Section 3. JSX
JSX의 정의와 역할
- A syntax extension to JavaScript
- JavaScript + XML/HTML
- 내부적으로 XML/HTML 코드를 JavaScript 코드로 변환하는 역할을 함
- JSX를 사용하면 react에서는 내부적으로 createElement 함수를 사용하도록 변환 됨
JSX의 장점 및 사용법
- 간결
- 가독성 향상
- 버그 발견 쉬움
- Injection Attacks 방어
- XML/HTML 코드 사이에 { } 를 사용해서 js 코드를 넣어주면 됨
(실습)JSX 코드 작성해보기
import React from "react";
function Book(props) {
return (
<div>
<h1>{`이 책의 이름은 ${props.name} 입니다.`}</h1>
<h2>{`이 책은 총 ${props.numOfPage}페이지로 이뤄져 있습니다.`}</h2>
</div>
);
}
export default Book;
import React from "react";
import Book from "./Book";
function Library(props) {
return (
<div>
<Book name="처음 만난 파이썬" numOfPage={300} />
<Book name="처음 만난 AWS" numOfPage={400} />
<Book name="처음 만난 리액트" numOfPage={500} />
</div>
);
}
export default Library;
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import Library from './chapter_03/Library';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<Library />
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
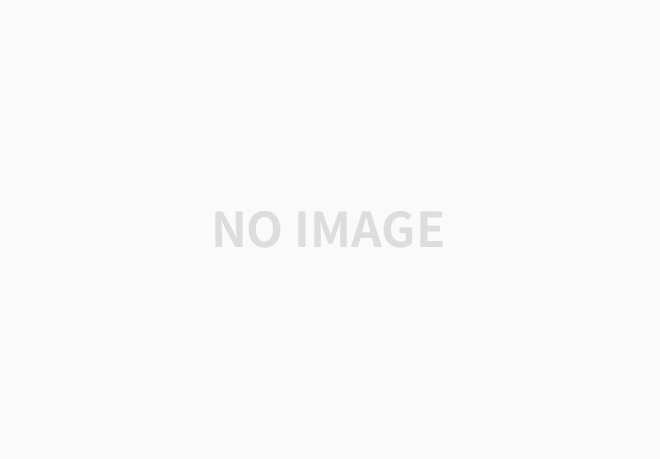
Section 4. Rendering Elements
Elements의 정의와 생김새
- 엘리먼트: 리액트 앱을 구성하는 가장 작은 블록들
- React Elements vs DOM Elements : react elements 는 virtual. DOM element의 가상표현이라고 보면 됨
- Elements는 화면에서 보이는 것을 기술
- react elements는 자바스크립트 객체 형태로 존재
Elements의 특징 및 렌더링하기
- immutable: 불변성. 한 번 생성된 elemet는 수정할 수 없다.
- elements 생성 후에는 childeren이나 attribute를 수정할 수 없다.
- 페이지를 변경하려면 새로운 elemets를 만들어서 기존의 elemets랑 바꿔치기 하면 됨
- elements 렌더링: react element를 DOM element에 렌더링, virtual DOM에서 실제 DOM으로 이동하는 과정이라고 생각하면 됨
(실습)시계 만들기
import React from "react";
function Clock(props) {
return (
<div>
<h1>안녕 리액트^_~</h1>
<h2>현재 시간: {new Date().toLocaleTimeString()}</h2>
</div>
);
}
export default Clock;
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import Library from './chapter_03/Library';
import Clock from './chapter_04/Clock';
const root = ReactDOM.createRoot(document.getElementById('root'));
setInterval(() => {
root.render(
<React.StrictMode>
<Clock />
</React.StrictMode>
);
}, 1000);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
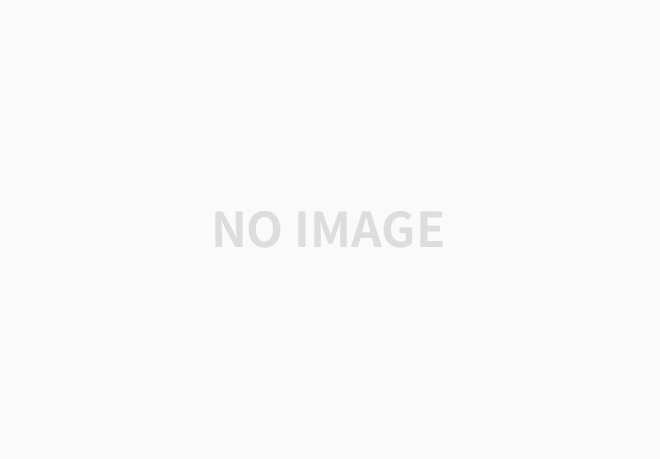
- setInterval로 1000ms마다 Clock 컴포넌트 엘리먼트를 새로 생성하도록 설정
Section 5. Components and Props
Components와 Props의 정의
components
- 리액트는 작은 컴포넌트들이 모여 하나의 컴포넌트를 구성하고 또 그 컴포넌트들이 모여 전체 페이지를 구성하고..
- 리액트 컴포넌트를 하나의 함수라고 생각하면 쉬움
props
- react component의 입력은 Props. 어떠한 속성들. 이를 입력받아 그에 맞는 react element를 생성해서 돌려주게 됨
- Props는 react component의 속성. 붕어빵에 들어가는 재료. 컴포넌트에 전달할 다양한 정보를 담고 있는 자바스크랩트 객체.
Props의 특징 및 사용법
- RDONLY. 값을 변경할 수 없다.
- 붕어빵 다 굽고 나서 속재료 바꿀 수 없는 거랑 똑같
- 새로운 값을 컴포넌트에 전달하여 새로 element를 만드는 수밖에 없음
pure vs impure
- pure: input 변경X. 똑같은 input에 대해 항상 같은 output을 리턴하는 경우.
- impure: input 변경하는 경우
- 모든 리액트 컴포넌트는 Props를 직접 바꿀 수 없고, 같은 Props에 대해서 항상 같은 결과를 보여줄 것! == pure해야 한다.
Props 사용법
- jsx를 사용하는 경우, key=value 식으로 사용
- 중괄호를 사용해 java script 사용 가능
Component 만들기 및 렌더링
- function component vs class component
- class component의 가장 큰 차이점은 React.Component를 상속받아 만든다는 것
- Component 이름은 항상 대문자로 시작해야 한다!
- React는 소문자로 시작하는 컴포넌트들은 DOM 태그로 인식한다.
Component 합성과 추출
- 여러 개의 컴포넌트 합쳐서 하나의 컴포넌트 생성 가능
- 복잡한 컴포넌트를 쪼개서 여러 개의 컴포넌트로 사용가능
- 재사용성 up 개발속도 up
- 컴포넌트 추출 기준은 없지만 재사용 기준으로 컴포넌트를 추출하는 것이 좋음
(실습)댓글 컴포넌트 만들기
댓글 컴포넌트에 스타일 입히기
Comment 컴포넌트에 Props 추가하기
import React from "react";
const styles = {
wrapper: {
margin: 8,
padding: 8,
display: "flex",
flexDirection: "row",
border: "1px solid grey",
borderRadius: 16,
},
imageContainer: {},
image: {
width: 50,
height: 50,
borderRadius: 25,
},
contentContainer: {
marginLeft: 8,
display: "flex",
flexDirection: "column",
justifyContent: "center",
},
nameText: {
color: "black",
fontSize: 16,
fontWeight: "bold",
},
commentText: {
color: "black",
fontSize: 16,
},
};
function Comment(props) {
return (
<div style={styles.wrapper}>
<div style={styles.imageContainer}>
<img
src="https://w7.pngwing.com/pngs/785/675/png-transparent-potato-illustration-baked-potato-potato-wedges-salt-potatoes-baked-beans-potato-high-quality-computer-network-food-performance.png"
style={styles.image}
/>
</div>
<div style={styles.contentContainer}>
<span style={styles.nameText}>{props.name}</span>
<span style={styles.commentText}>{props.comment}</span>
</div>
</div>
);
}
export default Comment;
import React from "react";
import Comment from "./Comment"
function CommentList(props) {
return (
<div>
<Comment name={"오지현"} comment={"제가 만든 첫 컴포넌트임다."}/>
</div>
);
}
export default CommentList;
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import Library from './chapter_03/Library';
import Clock from './chapter_04/Clock';
import CommentList from './chapter_05/CommentList';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<CommentList />
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
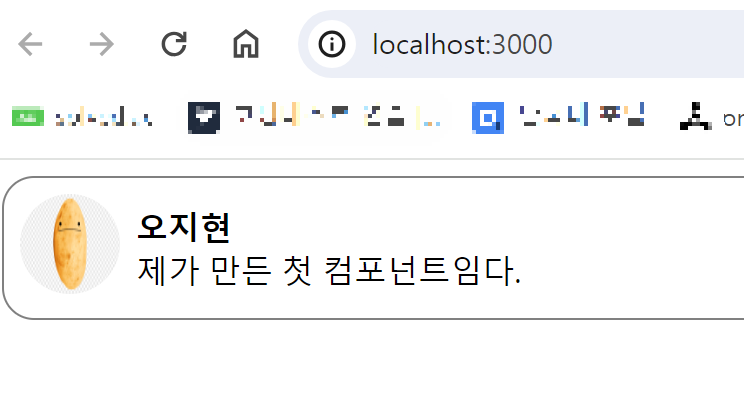
Comment 데이터를 별도의 객체로 분리하기
import React from "react";
const styles = {
wrapper: {
margin: 8,
padding: 8,
display: "flex",
flexDirection: "row",
border: "1px solid grey",
borderRadius: 16,
},
imageContainer: {},
image: {
width: 50,
height: 50,
borderRadius: 25,
},
contentContainer: {
marginLeft: 8,
display: "flex",
flexDirection: "column",
justifyContent: "center",
},
nameText: {
color: "black",
fontSize: 16,
fontWeight: "bold",
},
commentText: {
color: "black",
fontSize: 16,
},
};
function Comment(props) {
return (
<div style={styles.wrapper}>
<div style={styles.imageContainer}>
<img
src="https://w7.pngwing.com/pngs/785/675/png-transparent-potato-illustration-baked-potato-potato-wedges-salt-potatoes-baked-beans-potato-high-quality-computer-network-food-performance.png"
style={styles.image}
/>
</div>
<div style={styles.contentContainer}>
<span style={styles.nameText}>{props.name}</span>
<span style={styles.commentText}>{props.comment}</span>
</div>
</div>
);
}
export default Comment;
import React from "react";
import Comment from "./Comment"
const comments = [
{
name: "오지현",
comment: "안녕하세요, 오지현입니다.",
},
{
name: "이민형",
comment: "I'm a child",
},
{
name: "이장원",
comment: "캔 위 메이컵 인 더 모닝",
},
{
name: "익스",
comment: "잘 부탁드립니다",
},
];
function CommentList(props) {
return (
<div>
{comments.map((comment) => {
return (
<Comment name={comment.name} comment={comment.comment} />
);
})}
</div>
);
}
export default CommentList;
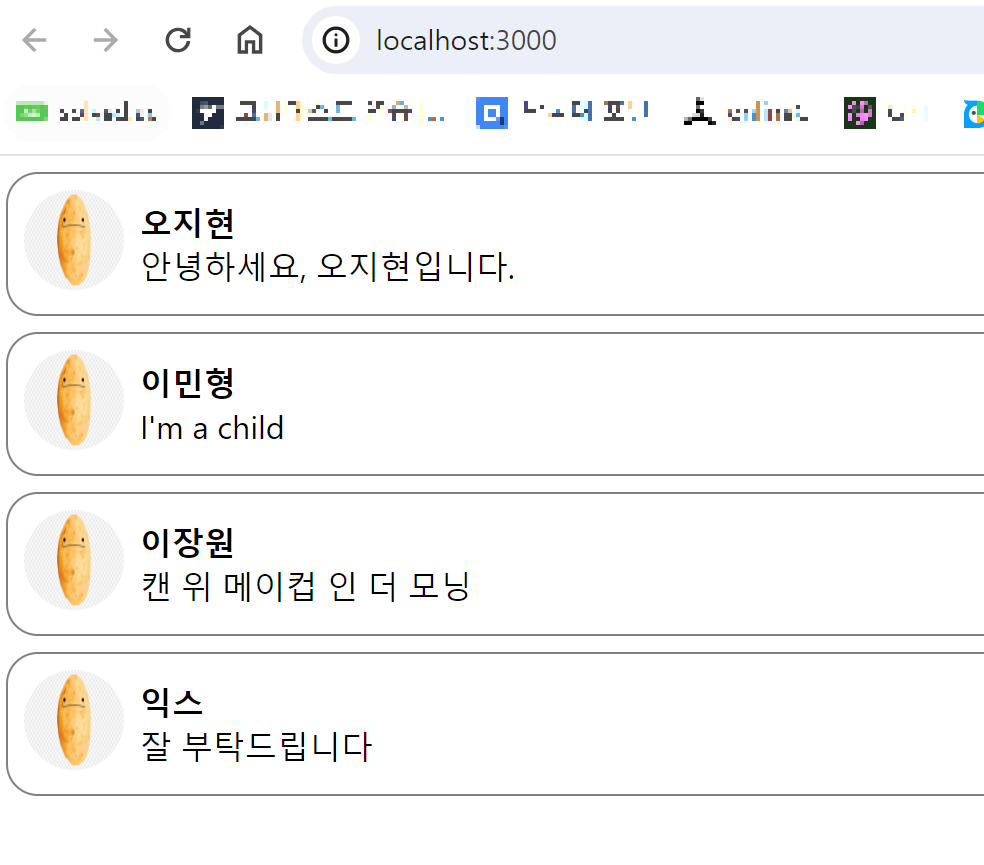
'Develop > React' 카테고리의 다른 글
[React] 처음 만난 리액트 section(0~2) (0) | 2024.05.18 |
---|
댓글